[M1 Mac, Monterey 12.6.3, clang 13.0.0, SDL 2.26.2, ChatGPT Plus, NO IDE]
テトリスをプレイ中に暫定順位(画面左下のCurrent Rank)を表示するようにしました。
これでベストテン入りにどれくらい近づいているかが分かるようになります。
ゲームアプリでは時間軸というファクターが加わり繰り返し演算し続けるため、これに巻き込まれないように計算しないと変数が知らぬ間に加算されたりします。
C++版の完成度が上がってきたので、そろそろiOS版の製作に着手します。
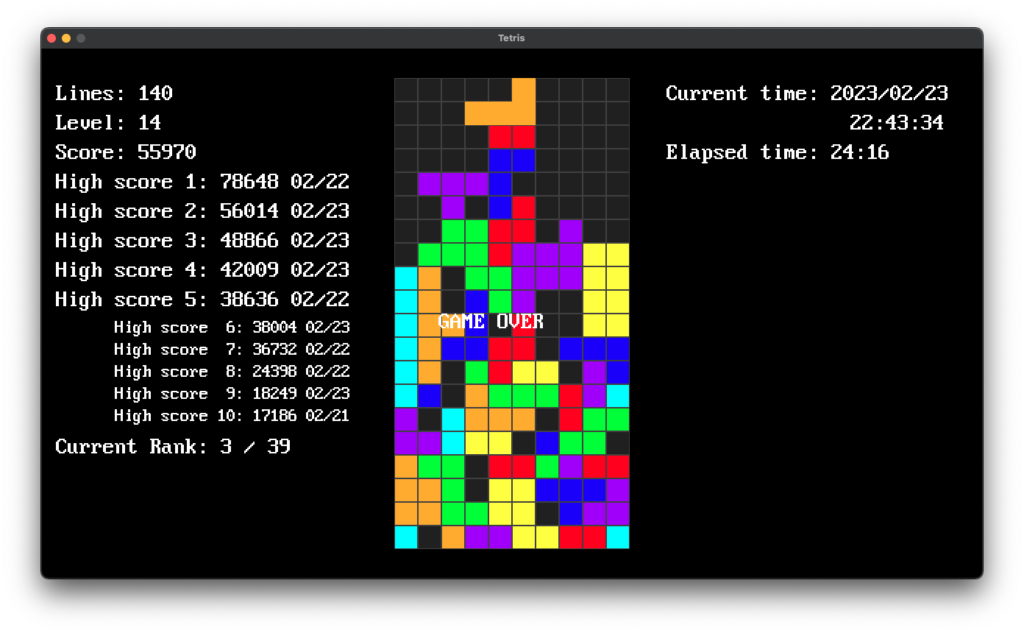
int m_score;
int numAllScores;
int rank;
vector<int> scores;
vector<int> allScores;
vector<string> dates;
vector<string> times;
struct Entry {
int score;
string date;
string playTime;
};
void makeRanking(){
// 暫定順位算出
allScores.push_back(m_score);
sort(allScores.begin(), allScores.end(), std::greater<int>());
numAllScores = allScores.size();
for (int i = 0; i < allScores.size(); ++i) {
if (allScores[i] == m_score) {
rank = i + 1;
printf("現在順位: %d / %d\n", rank, numAllScores);
break;
}
++rank;
}
allScores.pop_back();
}
int TetroTypeGenerator()
{
int tetroType;
if (GeneratorCount == 0){
arr = {0, 1, 2, 3, 4, 5, 6};
}
while(1){
tetroType = rand() % kNumTetrominoTypes;
if (arr.size() == 0){
arr = {0, 1, 2, 3, 4, 5, 6};
cout << "テトロミノ配列が空になったのでリセットしました" << endl;
} else if (arr.size() == 1){
tetroType = arr.front();
arr = {};
cout << "tetroType残り1: " << to_string(tetroType) << endl;
makeRanking();
GeneratorCount++;
return tetroType;
}
for (auto it = arr.begin(); it != arr.end(); ) {
if (*it == tetroType) {
it = arr.erase(it);
cout << "tetroType: " << to_string(tetroType) << endl;
makeRanking();
GeneratorCount++;
return tetroType;
} else {
it++;
}
}
}
}
int GetScoreAndDate() {
vector<Entry> entries = ReadCSV(csvFile);
allScores = {};
// スコアで降順ソート
sort(entries.begin(), entries.end(),
[](const Entry& a, const Entry& b) { return a.score > b.score; });
// 全スコアのvector作成
for (const auto& entry : entries) {
allScores.push_back(entry.score);
// cout << entry.score << " : " << entry.date << " : " << entry.playTime << std::endl;
}
// 上位10件を取得して、スコアと日付をvectorに格納
for (int i = 0; i < std::min(10, static_cast<int>(entries.size())); ++i) {
scores.push_back(entries[i].score);
string date = entries[i].date;
replace(date.begin(), date.end(), '-', '/');
dates.push_back(date.substr(5, 5));
}
return 0;
}
void Game::DrawPlaying( Renderer& renderer )
{
<中略>
snprintf( text, sizeof(text), "Current Rank: %s / %s", to_string(rank).c_str(), to_string(numAllScores).c_str());
renderer.DrawText( text, 20, 530, mFont, 0xffffffff);
<以下略>
}