[M1 Mac, Monterey 12.6.3, clang 13.0.0, SDL 2.26.2, ChatGPT Plus, NO IDE]
スタート画面でテトリスの落下速度を選べるようにしました。今の私のゲームスキルでSlowは20分、Normalは10分、Fastは5分程度でケリがつくように調整しました。
PS4コントローラーのR1ボタンかマウスクリックで選択できます。
selectButton関数の叩きはChatGPTが書いてくれました。
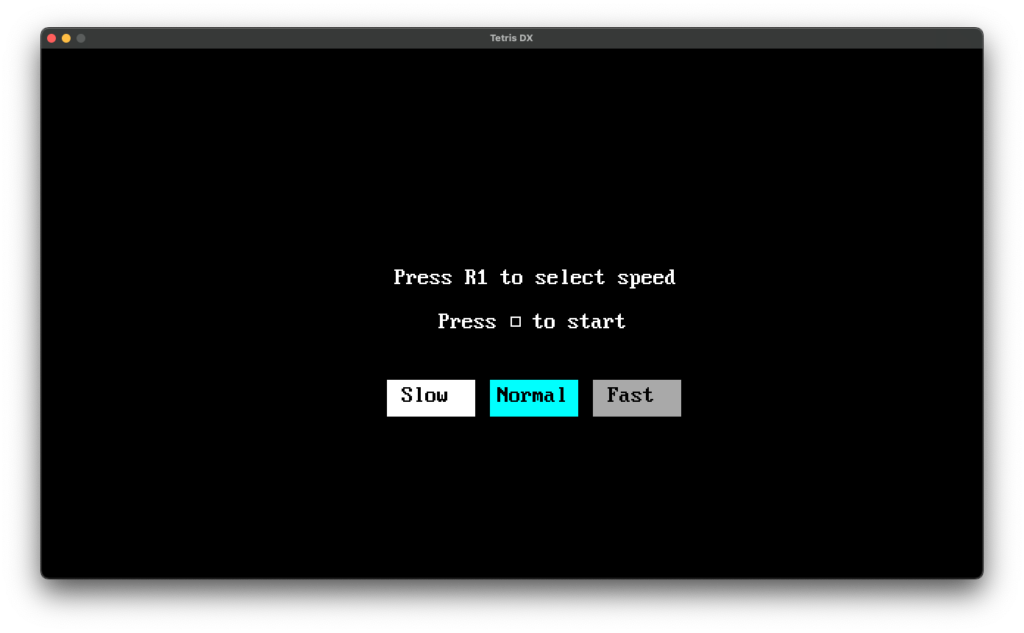
void App::Run()
{
Uint32 lastTimeMs = SDL_GetTicks();
auto lastTime = std::chrono::high_resolution_clock::now();
bool bDone = false;
while( !bDone )
{
GameInput gameInput = {};
SDL_JoystickUpdate();
// respond to events
SDL_Event event;
while( SDL_PollEvent( &event ) )
{
if(event.type == SDL_QUIT)
{
bDone = true;
}
// 落下速度ボタン選択(マウスクリック)
if(event.type == SDL_MOUSEBUTTONDOWN)
{
mouseX = event.motion.x;
mouseY = event.motion.y;
SDL_Delay(10);
selectButton(slowButton, normalButton, fastButton, mouseX, mouseY);
}
if(event.type == SDL_JOYBUTTONDOWN)
{
switch (event.jbutton.button){
case 2:{ // □ボタン:スタート
gameInput.bStart = true;
start = std::chrono::steady_clock::now();
scores = {};
dates = {};
fullDates = {};
numAllScores = {};
rank = 0;
DrawPlayingCount = 0;
GeneratorCount = 0;
arr = {};
break;
}
case 13:{ // 左ボタン
gameInput.bMoveLeft = true;
break;
}
case 14:{ // 右ボタン
gameInput.bMoveRight = true;
break;
}
case 1:{ // ○ボタン:反時計回り回転
gameInput.bRotateClockwise = true;
break;
}
case 0:{ // ×ボタン:時計回り回転
gameInput.bRotateAnticlockwise = true;
break;
}
case 12:{ // 下ボタン:一気に落下
gameInput.bHardDrop = true;
break;
}
case 11:{ // 上ボタン:徐々に落下
gameInput.bSoftDrop = true;
break;
}
case 10:{ // R1ボタン 落下速度ボタン選択
buttonCount++;
if (buttonCount % 3 == 1){
fastButton.selected = true;
normalButton.selected = false;
s_initialFramesPerFallStep = 24;
} else if (buttonCount % 3 == 2){
slowButton.selected = true;
fastButton.selected = false;
s_initialFramesPerFallStep = 48;
} else {
normalButton.selected = true;
slowButton.selected = false;
s_initialFramesPerFallStep = 32;
}
break;
}
default:{
cout << "default" << endl;
break;
}
}
}
}
// FPS算出のために1フレーム時間を計算
Uint32 currentTimeMs = SDL_GetTicks();
Uint32 deltaTimeMs = currentTimeMs - lastTimeMs;
lastTimeMs = currentTimeMs;
HP_UNUSED( deltaTimeMs );
auto currentTime = std::chrono::high_resolution_clock::now();
auto deltaTime = currentTime - lastTime;
std::chrono::microseconds deltaTimeMicroseconds = std::chrono::duration_cast<std::chrono::microseconds>(deltaTime);
float deltaTimeSeconds = 0.000001f * (float)deltaTimeMicroseconds.count();
lastTime = currentTime;
mGame->Update(gameInput, deltaTimeSeconds );
mRenderer->Clear();
mGame->Draw(*mRenderer );
mRenderer->Present();
}
}
void selectButton(Button& slow, Button& normal, Button& fast, const int mouseX, const int mouseY) {
slow.selected = false;
normal.selected = false;
fast.selected = false;
if (mouseX >= slow.rect.x && mouseX <= slow.rect.x + slow.rect.w &&
mouseY >= slow.rect.y && mouseY <= slow.rect.y + slow.rect.h) {
slow.selected = true;
s_initialFramesPerFallStep = 48;
cout << "slow clicked" << endl;
} else if (mouseX >= normal.rect.x && mouseX <= normal.rect.x + normal.rect.w &&
mouseY >= normal.rect.y && mouseY <= normal.rect.y + normal.rect.h) {
normal.selected = true;
s_initialFramesPerFallStep = 32;
cout << "normal clicked" << endl;
} else if (mouseX >= fast.rect.x && mouseX <= fast.rect.x + fast.rect.w &&
mouseY >= fast.rect.y && mouseY <= fast.rect.y + fast.rect.h) {
fast.selected = true;
s_initialFramesPerFallStep = 24;
cout << "fast clicked" << endl;
}
}