[M1 Mac, Monterey 12.6.3, clang 13.0.0, FLTK 1.3.8, ChatGPT Plus, NO IDE]
jsonデータのパースエラーを捕捉するtry-catch文をメモ書きしておきます。
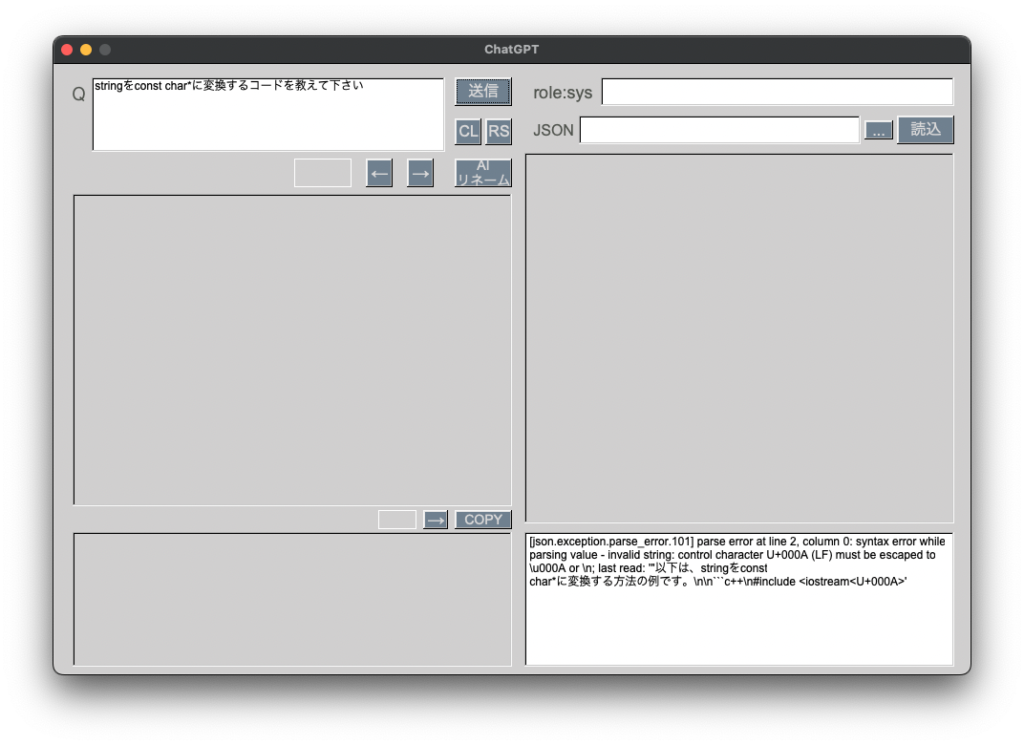
try{
resJson = json::parse(res);
}
catch (const json::parse_error& e) {
std::cerr << "Parse error:\n" << e.what() << std::endl;
Fl_Text_Buffer* bufferError = new Fl_Text_Buffer();
bufferError -> text(e.what());
noticeDisplay->buffer(bufferError);
noticeDisplay -> wrap_mode(Fl_Text_Display::WRAP_AT_BOUNDS, 5);
return;
}
catch (const char* error) {
cerr << "Error: " << error << endl;
Fl_Text_Buffer* bufferError = new Fl_Text_Buffer();
bufferError -> text(error);
noticeDisplay->buffer(bufferError);
noticeDisplay -> wrap_mode(Fl_Text_Display::WRAP_AT_BOUNDS, 5);
return;
}
catch (...){
cerr << "その他のError" << endl;
const char* error = "その他のError";
Fl_Text_Buffer* bufferError = new Fl_Text_Buffer();
bufferError -> text(error);
noticeDisplay->buffer(bufferError);
noticeDisplay -> wrap_mode(Fl_Text_Display::WRAP_AT_BOUNDS, 5);
return;
}