[M1 Mac, Monterey 12.6.3, clang 13.0.0, FLTK 1.3.8, ChatGPT Plus, NO IDE]
AIリネーム機能を改良しました。半角スペースやカギ括弧を削除するための関数を作成しました。この関数は今後も重宝しそうです。
こういった気の利いた関数をChatGPTに書かせようとすると高確率でデタラメを提示してくるので、最初から自分で書く方が速かったりします。完成目前の仕上げはしっかりやってくれることもあります。
ここまで機能を揃えるともうChatGPT Plusを解約しても問題ないでしょう。来週は月更新せずに済みそうです。
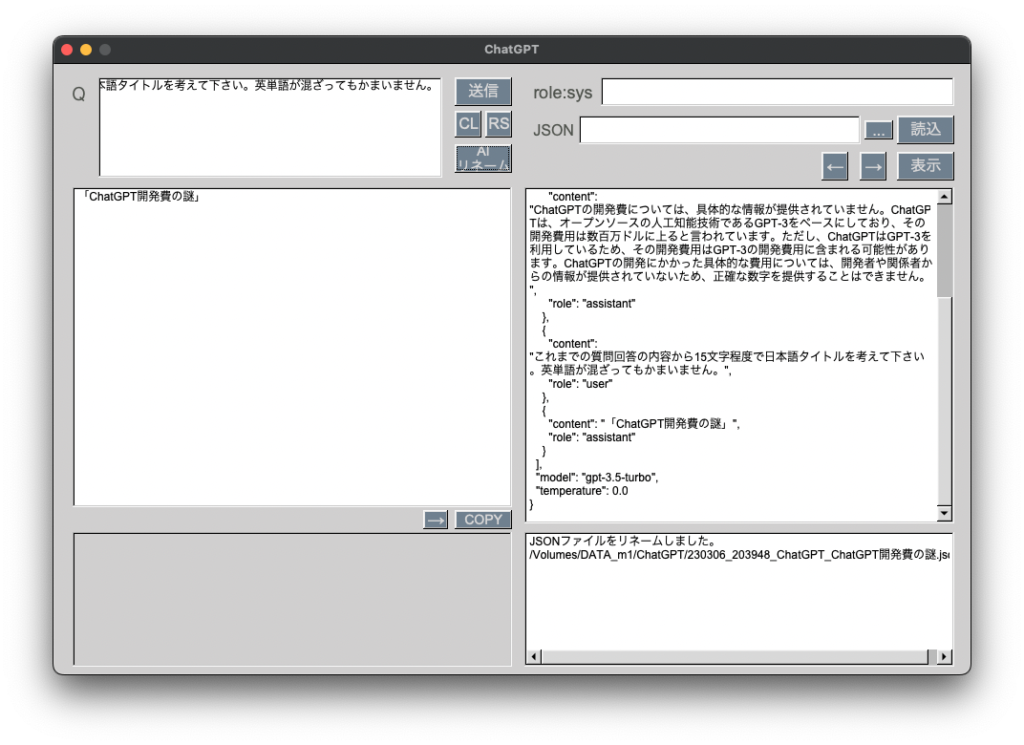
void deleteString(string& str, const char* del) {
size_t size = strlen(del);
size_t pos = 0;
while ((pos = str.find(del, pos)) != string::npos) {
// cout << "pos: " << pos << endl;
str.erase(pos, size);
}
}
void renameCB(Fl_Widget*, void*){
input -> value("これまでの質問回答の内容から15文字程度で日本語タイトルを考えて下さい。英単語が混ざってもかまいません。");
sendBtn->do_callback();
Fl_Text_Buffer* buffer = output->buffer();
title = buffer->text();
cout << "title加工前: " << title << endl;
// 半角スペースと「」を削除
deleteString(title, " ");
deleteString(title, "「");
deleteString(title, "」");
cout << "title加工後: " << title << endl;
vector<string> tokens;
string delimiter = ".";
size_t pos = 0;
string token;
string jsonFileStr = string(jsonFile);
while ((pos = jsonFileStr.find(delimiter)) != string::npos) {
token = jsonFileStr.substr(0, pos);
tokens.push_back(token);
jsonFileStr.erase(0, pos + delimiter.length());
}
tokens.push_back(jsonFile);
string jsonFileNew = tokens[0] + "_" + title + ".json";
cout << "jsonFileNew: " << jsonFileNew << endl;
int result = std::rename(jsonFile.c_str(), jsonFileNew.c_str());
if (result == 0) {
std::printf("File renamed successfully.\n");
} else {
std::printf("Error renaming file.\n");
}
Fl_Text_Buffer* bufferNotice = new Fl_Text_Buffer();
string notice = "JSONファイルをリネームしました。\n" + jsonFileNew;
bufferNotice -> text(notice.c_str());
noticeDisplay -> buffer(bufferNotice);
}