[M1 Mac, Big Sur 11.7.2, Python 3.10.4, MySQL 8.0.31]
MySQL高速化のため非Webサーバ環境でデータベースを作成してみました。
その結果、テーブルの作成時間を3割削減できました。
クエリやテーブルの最適化など他の方法についても試していきます。
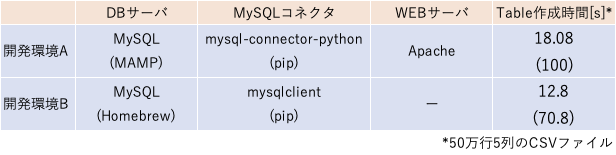
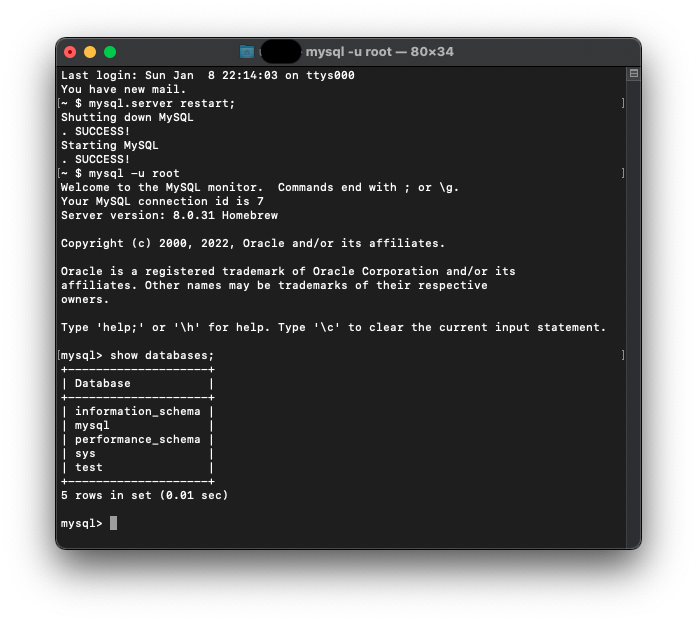
import MySQLdb
import pandas as pd
import time,datetime,glob,csv
start = time.time()
num = 1
for file in glob.glob(f'/*.csv'):
# CSVファイル名を抽出しtable名にする
tablename = file[-12:-4]
# print(tablename)
column_title = ['A', 'B', 'C', 'D', 'E']
column_type = ['varchar(256)','varchar(512)','varchar(512)','int(9)','int(8)']
# SQL文に使う列名&データ型の文字列を作成する
column_l = []
for ti,ty in zip(column_title,column_type):
column = ti + ' ' + str(ty)
column_l.append(column)
# print(column_l)
# SQL仕様に整形
column_l_str = str(column_l).replace('[','(').replace(']',')').replace("'",'')
# print(column_l_str)
# MySQLに接続
conn = MySQLdb.connect(user='root')
cur = conn.cursor()
# データベースdataにtableを作成する
sql = f"create table test.{tablename} {column_l_str} CHARACTER SET utf8mb4"
cur.execute(sql)
cur.execute('begin')
# CSVファイルを読み込み、各行をtableに挿入する
with open(file, 'rt', encoding='UTF-8') as f:
reader = csv.reader(f)
for i,row in enumerate(reader):
# print(f'row {row}')
if i != 0:
row_str = str(row).replace('[','(').replace(']',')')
# print(f'{i+1}:{row_str}')
sql = f'insert into test.{tablename} values {row_str}'
cur.execute(sql)
cur.execute('commit')
conn.close()
# 処理時間算出(秒)
process_time = time.time() - start
td = datetime.timedelta(seconds = process_time).total_seconds()
# 小数点第2位まで表示
td_2f = f'{td:.2f}'
print(f'{num}:{td_2f}')
num = num + 1