nkfコマンドを使って仕上げました。
jar実行ファイルではこれまでと同じく文字コード判定ができませんでしたが、標準エラー出力をチェックしたところ、nkfコマンドを見つけられないことが判明しました。
nkfファイルは/usr/binフォルダには入っておらず、絶対パスのコマンドにすると解決しました。jar実行ファイルではnkfファイルへのpathが通らなくなるようです。
Pythonのtkinterでもコンソールなしの実行ファイルではうまく動作しないケースがあるのですが、コマンドの書き方がおかしいのかもしれません。
とりあえずこれで完成です。UniversalDetectorを使ったJavaオリジナルのコードも書いてみたいです。
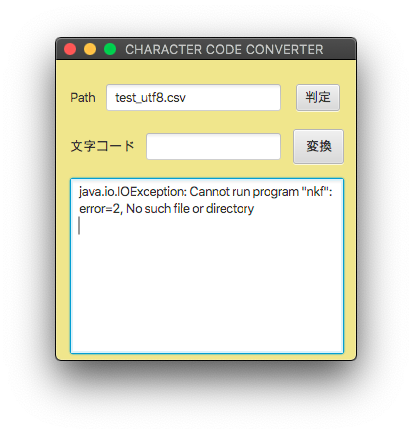
package com.example;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.control.TextArea;
import javafx.event.ActionEvent;
import java.util.regex.Pattern;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStreamReader;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileOutputStream;
import java.io.OutputStreamWriter;
public class GuiController {
@FXML
private Button button1;
@FXML
private Button button2;
@FXML
private TextField text1;
@FXML
private TextField text2;
@FXML
private TextArea text3;
String path;
String path2;
String code;
String code2;
String str1;
@FXML
void onButton1Action(ActionEvent event) {
code2 = text2.getText();
path2 = text1.getText();
if(code2.matches("UTF-8")){
// 変換後のファイル名を作成
StringBuffer buf = new StringBuffer();
buf.append(path2.split(Pattern.quote("."))[0]);
buf.append("_s.");
buf.append(path2.split(Pattern.quote("."))[1]); // 拡張子
String s = buf.toString();
// ファイルの文字コードをUTF-8からシフトJISに変換
File file = new File(path2);
File file2 = new File(s);
try (BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream(file),"UTF-8"));BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(file2),"Shift-JIS"))){
String line;
while ((line = br.readLine()) != null) {
bw.write(line);
bw.newLine();
}
}
catch (Exception e) {
e.printStackTrace();
}
}
else{
if(code2.matches("SHIFT_JIS")){
// 変換後のファイル名を作成
StringBuffer buf = new StringBuffer();
buf.append(path2.split(Pattern.quote("."))[0]);
buf.append("_u.");
buf.append(path2.split(Pattern.quote("."))[1]); // 拡張子
String s = buf.toString();
// ファイルの文字コードをUTF-8からシフトJISに変換
File file = new File(path2);
File file2 = new File(s);
try (BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream(file),"Shift-JIS"));BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(file2),"UTF-8"))){
String line;
while ((line = br.readLine()) != null) {
bw.write(line);
bw.newLine();
}
}
catch (Exception e) {
e.printStackTrace();
}
}
else{
text2.setText("このツールでは不可");
}
}
}
@FXML
void onButton2Action(ActionEvent event) {
path = text1.getText();
try{
Function fc = new Function();
str1 = fc.main(path);
text3.appendText(str1);
}
catch (Exception e){
System.out.println(e);
text3.appendText("Function失敗\n");
}
if (str1 != null){
text2.appendText(str1 + "\n");
}
else{
text3.appendText("判定不可\n");
}
}
}
package com.example;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.nio.charset.Charset;
public class Function {
StringBuffer buf = new StringBuffer();
public String main(String path) throws IOException {
ProcessBuilder p = new ProcessBuilder("/usr/local/Cellar/nkf/2.1.5/bin/nkf","-g",path);
p.redirectErrorStream(true);
try{
Process process = p.start();
process.waitFor();
// 判定結果を受け取る
try (BufferedReader r = new BufferedReader(new InputStreamReader(process.getInputStream(), Charset.defaultCharset()))) {
String line;
int count = 1;
while ((line = r.readLine()) != null) {
if(count == 1){
buf.append(line + "\n");
}
else{
break;
}
count = count + 1;
}
}
}
catch (Exception e) {
buf.append(e + "\n");
}
String s = buf.toString();
return s;
}
}