一応完成しました。
昨年2020年9月にGUIアプリではないですがPython&Bashで同じことができるコードを書いています。nkfコマンドを使えばファイルの文字コード変換は楽勝です。
こちらの方の難易度は桁違いに高かったです。
あとはjar実行ファイルに変換して普段使いできるようにします。
先月下旬にVScodeでのJavaツール開発を諦めてEclipseへ移行してから1ヶ月余り。ようやくVScodeでも開発できるようになりました。XcodeやEclipseのようなIDEはなにか窮屈で書かされている感もあり苦手です。
package javafx_conv;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.event.ActionEvent;
import java.util.regex.Pattern;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStreamReader;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileOutputStream;
import java.io.OutputStreamWriter;
public class GuiController {
@FXML
private Button button1;
@FXML
private Button button2;
@FXML
private TextField text1;
@FXML
private TextField text2;
String path;
String path2;
String code;
String code2;
@FXML
void onButton1Action(ActionEvent event) {
code2 = text2.getText();
System.out.println(code2);
path2 = text1.getText();
System.out.println(path2);
if(code2.matches("UTF-8")){
// 変換後のファイル名を作成
StringBuffer buf = new StringBuffer();
buf.append(path2.split(Pattern.quote("."))[0]);
buf.append("_s.");
buf.append(path2.split(Pattern.quote("."))[1]); // 拡張子
String s = buf.toString();
System.out.println(s);
// ファイルの文字コードをUTF-8からシフトJISに変換
File file = new File(path2);
File file2 = new File(s);
try (BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream(file),"UTF-8"));BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(file2),"Shift-JIS"))){
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
bw.write(line);
bw.newLine();
}
}
catch (Exception e) {
e.printStackTrace();
}
}
else{
if(code2.matches("SHIFT_JIS")){
// 変換後のファイル名を作成
StringBuffer buf = new StringBuffer();
buf.append(path2.split(Pattern.quote("."))[0]);
buf.append("_u.");
buf.append(path2.split(Pattern.quote("."))[1]); // 拡張子
String s = buf.toString();
System.out.println(s);
// ファイルの文字コードをシフトJISからUTF-8に変換
File file = new File(path2);
File file2 = new File(s);
try (BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream(file),"Shift-JIS"));BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(file2),"UTF-8"))){
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
bw.write(line);
bw.newLine();
}
}
catch (Exception e) {
e.printStackTrace();
}
}
else{
text2.setText("");
text2.setText("このツールでは不可");
}
}
}
@FXML
void onButton2Action(ActionEvent event) {
path = text1.getText();
FileCharDetector fd = new FileCharDetector(path);
try{
code = fd.detector();
}
catch (Exception e) {
System.out.println(e);
}
if (code != null){
text2.setText(code);
}
else{
text2.setText("判定不可");
}
}
}
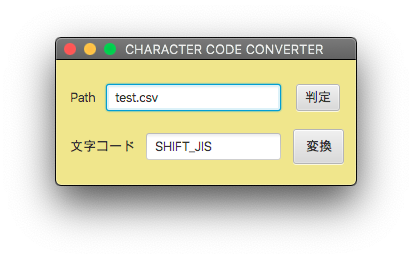