以前うまくいかなかったC言語モジュールを完成させました。
input(引数)とoutput(戻り値)を自在に設定でき、C言語実行ファイルに必須の処理終了検知が不要なので、こちらを常用することになりそうです。
#define PY_SSIZE_T_CLEAN
#include <Python.h>
#include "fnv_function.c"
static PyObject* horse_id(PyObject* self, PyObject* args)
{
const char* path;
const char* name;
uint32_t id;
if (!PyArg_ParseTuple(args,"ss",&path,&name)){
return NULL;
}
else{
FILE *fp; // horse_listファイル
int horseID[10]; // 1 horseID
uint32_t horse_hash[20]; // 2 馬名ハッシュ
char horse_name[100]; // 3 検索馬名
char horse_name0[100]; // 4 馬名
char status[10]; // 5 稼働
char gender[10]; // 6 性別
char hair[10]; // 7 毛色
char birthday[100]; // 8 生年月日
char trainer[100]; // 9 調教師
char owner[100]; // 10 馬主
char info[100]; // 11 募集情報
char breeder[100]; // 12 生産者
char area[50]; // 13 産地
char price[50]; // 14 セリ取引価格
char prize_money[50]; // 15 獲得賞金
char result[50]; // 16 通算成績
char wining_race[200]; // 17 主な勝鞍
char relatives[200]; // 18 近親馬
uint32_t horse_hash_input_int;
uint32_t horse_hash_int;
char buf[2000]; // fgets用
int i=0; // 行番号
int b=0; // 検索結果有無の識別
fp = fopen(path, "r");
while(fgets(buf,2000,fp ) != NULL ) {
if (i != 0){
sscanf(buf, " %[^,],%[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %[^,], %s",horseID,horse_hash,horse_name,horse_name0,status,gender,hair,birthday,trainer,owner,info,breeder,area,price,prize_money,result,wining_race,relatives) ;
horse_hash_int = atoi(horse_hash);
horse_hash_input_int = fnv_1_hash_32(name);
if(horse_hash_int - horse_hash_input_int == 0){
id = atoi(horseID);
b ++;
break;
}
}
i ++ ;
}
if (b == 0){
id = 100000000;
}
fclose(fp);
return Py_BuildValue("I", id);
}
}
static PyMethodDef Horseidmethods[] = {
{"horse_id", (PyCFunction)horse_id, METH_VARARGS},
{NULL,NULL,0}
};
static struct PyModuleDef horseid = {
PyModuleDef_HEAD_INIT,
"horseid",
"Python3 C API Module(Sample 1)",
-1,
Horseidmethods
};
PyMODINIT_FUNC PyInit_horseid(void)
{
return PyModule_Create(&horseid);
}
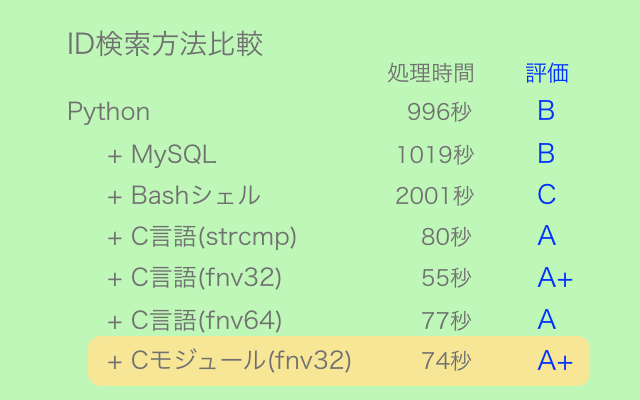