libpngを使ってpngファイルの縦横サイズと解像度のデータを取得しました。
width, height, dpi_x, dpi_yの値を配列std::array<int,4>にして戻り値としています。main関数は省略します。
#include "png.h"
#include <array>
std::array<int,4> getInfoPNG(const char*);
#define SIGNATURE_NUM 8
#include "process_image.h"
#include <string>
FILE *fi;
unsigned int width;
unsigned int height;
unsigned int res_x;
unsigned int res_y;
unsigned int readSize;
png_structp png;
png_infop info;
png_byte signature[8];
std::array<int,4> getInfoPNG(const char* filename){
fi = fopen(filename, "rb");
readSize = fread(signature, 1, SIGNATURE_NUM, fi);
png = png_create_read_struct(PNG_LIBPNG_VER_STRING, NULL, NULL, NULL);
info = png_create_info_struct(png);
png_init_io(png, fi);
png_set_sig_bytes(png, readSize);
png_read_png(png, info, PNG_TRANSFORM_PACKING | PNG_TRANSFORM_STRIP_16, NULL);
width = png_get_image_width(png, info);
height = png_get_image_height(png, info);
res_x = png_get_x_pixels_per_inch(png,info);
res_y = png_get_y_pixels_per_inch(png,info);
png_destroy_read_struct(&png, &info, NULL);
fclose(fi);
return {(int)width,(int)height,(int)res_x,(int)res_y};
}
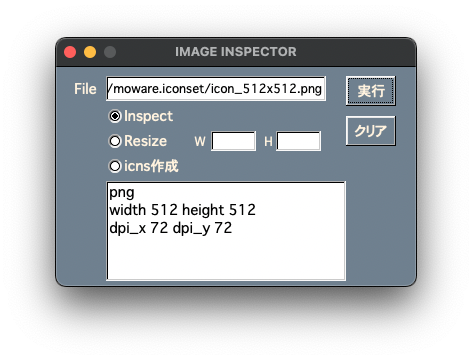